mirror of
https://github.com/ppy/osu.git
synced 2024-12-05 03:22:55 +08:00
Compare commits
10 Commits
a038a51a9b
...
0569443116
Author | SHA1 | Date | |
---|---|---|---|
|
0569443116 | ||
|
ce4aac4184 | ||
|
ee369ef86d | ||
|
9a4c419c56 | ||
|
3cfa455369 | ||
|
dbe2741982 | ||
|
83f8fa7472 | ||
|
9a89d402b9 | ||
|
2a7133d6d3 | ||
|
2417d4de83 |
@ -144,6 +144,13 @@ namespace osu.Game.Rulesets.Taiko.Difficulty
|
||||
foreach (var nested in hitObject.NestedHitObjects)
|
||||
simulateHit(nested, ref attributes);
|
||||
return;
|
||||
|
||||
case StrongNestedHitObject:
|
||||
// we never need to deal with these directly.
|
||||
// the only thing strong hits do in terms of scoring is double their object's score increase,
|
||||
// which is already handled at the parent object level via the `strongable.IsStrong` check lower down in this method.
|
||||
// not handling these here can lead to them falsely being counted as combo-increasing when handling strong drum rolls!
|
||||
return;
|
||||
}
|
||||
|
||||
if (hitObject is DrumRollTick tick)
|
||||
|
47
osu.Game.Tests/Visual/Online/TestSceneImageProxying.cs
Normal file
47
osu.Game.Tests/Visual/Online/TestSceneImageProxying.cs
Normal file
@ -0,0 +1,47 @@
|
||||
// Copyright (c) ppy Pty Ltd <contact@ppy.sh>. Licensed under the MIT Licence.
|
||||
// See the LICENCE file in the repository root for full licence text.
|
||||
|
||||
using NUnit.Framework;
|
||||
using osu.Framework.Allocation;
|
||||
using osu.Framework.Graphics;
|
||||
using osu.Framework.Platform;
|
||||
using osu.Game.Graphics.Containers.Markdown;
|
||||
|
||||
namespace osu.Game.Tests.Visual.Online
|
||||
{
|
||||
public partial class TestSceneImageProxying : OsuTestScene
|
||||
{
|
||||
[Resolved]
|
||||
private GameHost host { get; set; } = null!;
|
||||
|
||||
[Test]
|
||||
public void TestExternalImageLink()
|
||||
{
|
||||
AddStep("load image", () => Child = new OsuMarkdownContainer
|
||||
{
|
||||
RelativeSizeAxes = Axes.Both,
|
||||
Text = "",
|
||||
});
|
||||
}
|
||||
|
||||
[Test]
|
||||
public void TestLocalImageLink()
|
||||
{
|
||||
AddStep("load image", () => Child = new OsuMarkdownContainer
|
||||
{
|
||||
RelativeSizeAxes = Axes.Both,
|
||||
Text = "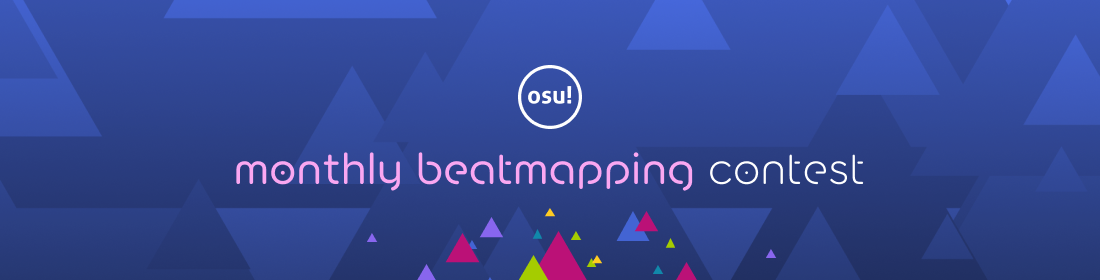",
|
||||
});
|
||||
}
|
||||
|
||||
[Test]
|
||||
public void TestInvalidImageLink()
|
||||
{
|
||||
AddStep("load image", () => Child = new OsuMarkdownContainer
|
||||
{
|
||||
RelativeSizeAxes = Axes.Both,
|
||||
Text = "",
|
||||
});
|
||||
}
|
||||
}
|
||||
}
|
@ -6,9 +6,10 @@ using osu.Framework.Audio;
|
||||
using osu.Framework.Audio.Track;
|
||||
using osu.Framework.Bindables;
|
||||
using osu.Framework.Graphics;
|
||||
using osu.Framework.IO.Stores;
|
||||
using osu.Framework.Logging;
|
||||
using osu.Game.Beatmaps;
|
||||
using osu.Game.Online;
|
||||
using osu.Game.Online.API;
|
||||
|
||||
namespace osu.Game.Audio
|
||||
{
|
||||
@ -28,9 +29,9 @@ namespace osu.Game.Audio
|
||||
}
|
||||
|
||||
[BackgroundDependencyLoader]
|
||||
private void load(AudioManager audioManager)
|
||||
private void load(AudioManager audioManager, IAPIProvider api)
|
||||
{
|
||||
trackStore = audioManager.GetTrackStore(new OnlineStore());
|
||||
trackStore = audioManager.GetTrackStore(new OsuOnlineStore(api.APIEndpointUrl));
|
||||
}
|
||||
|
||||
/// <summary>
|
||||
|
@ -17,5 +17,8 @@ namespace osu.Game.Graphics.Containers.Markdown
|
||||
{
|
||||
TooltipText = linkInline.Title;
|
||||
}
|
||||
|
||||
protected override ImageContainer CreateImageContainer(string url)
|
||||
=> base.CreateImageContainer($@"https://osu.ppy.sh/media-url?url={url}");
|
||||
}
|
||||
}
|
||||
|
31
osu.Game/Online/OsuOnlineStore.cs
Normal file
31
osu.Game/Online/OsuOnlineStore.cs
Normal file
@ -0,0 +1,31 @@
|
||||
// Copyright (c) ppy Pty Ltd <contact@ppy.sh>. Licensed under the MIT Licence.
|
||||
// See the LICENCE file in the repository root for full licence text.
|
||||
|
||||
using System;
|
||||
using osu.Framework.IO.Stores;
|
||||
using osu.Framework.Logging;
|
||||
|
||||
namespace osu.Game.Online
|
||||
{
|
||||
public class OsuOnlineStore : OnlineStore
|
||||
{
|
||||
private readonly string apiEndpointUrl;
|
||||
|
||||
public OsuOnlineStore(string apiEndpointUrl)
|
||||
{
|
||||
this.apiEndpointUrl = apiEndpointUrl;
|
||||
}
|
||||
|
||||
protected override string GetLookupUrl(string url)
|
||||
{
|
||||
// add leading dot to avoid matching hosts named "<anything>ppy.sh"
|
||||
if (!Uri.TryCreate(url, UriKind.Absolute, out Uri? uri) || !uri.Host.EndsWith(@".ppy.sh", StringComparison.OrdinalIgnoreCase))
|
||||
{
|
||||
Logger.Log($@"Blocking resource lookup from external website: {url}", LoggingTarget.Network, LogLevel.Important);
|
||||
return string.Empty;
|
||||
}
|
||||
|
||||
return url;
|
||||
}
|
||||
}
|
||||
}
|
@ -29,6 +29,7 @@ using osu.Framework.Input;
|
||||
using osu.Framework.Input.Bindings;
|
||||
using osu.Framework.Input.Events;
|
||||
using osu.Framework.Input.Handlers.Tablet;
|
||||
using osu.Framework.IO.Stores;
|
||||
using osu.Framework.Localisation;
|
||||
using osu.Framework.Logging;
|
||||
using osu.Framework.Platform;
|
||||
@ -824,6 +825,8 @@ namespace osu.Game
|
||||
|
||||
protected override Container CreateScalingContainer() => new ScalingContainer(ScalingMode.Everything);
|
||||
|
||||
protected override OnlineStore CreateOnlineStore() => new OsuOnlineStore(CreateEndpoints().APIEndpointUrl);
|
||||
|
||||
#region Beatmap progression
|
||||
|
||||
private void beatmapChanged(ValueChangedEvent<WorkingBeatmap> beatmap)
|
||||
|
@ -277,7 +277,7 @@ namespace osu.Game
|
||||
dependencies.CacheAs(Storage);
|
||||
|
||||
var largeStore = new LargeTextureStore(Host.Renderer, Host.CreateTextureLoaderStore(new NamespacedResourceStore<byte[]>(Resources, @"Textures")));
|
||||
largeStore.AddTextureSource(Host.CreateTextureLoaderStore(new OnlineStore()));
|
||||
largeStore.AddTextureSource(Host.CreateTextureLoaderStore(CreateOnlineStore()));
|
||||
dependencies.Cache(largeStore);
|
||||
|
||||
dependencies.CacheAs(LocalConfig);
|
||||
|
Loading…
Reference in New Issue
Block a user